PTSQ server
The ptsq
function creates an instance of the PTSQ server.
However, it does not create the server components themselves, it creates a builder that offers the ability to add middleware to the entire server in addition to creating components.
PTSQ itself does not offer any option to create an HTTP server. Like GraphQL or tRPC, PTSQ uses only a single HTTP endpoint. The library provides listeners for HTTP servers created by external modules such as node:http (opens in a new tab), Express (opens in a new tab), Fastify (opens in a new tab), or Koa (opens in a new tab), for serverless or alternative environments, such as Bun (opens in a new tab), Deno (opens in a new tab), AWS Lambda (opens in a new tab) or Cloudflare workers (opens in a new tab) and for full-stack frameworks, such as Next.js (opens in a new tab) or SvelteKit (opens in a new tab).
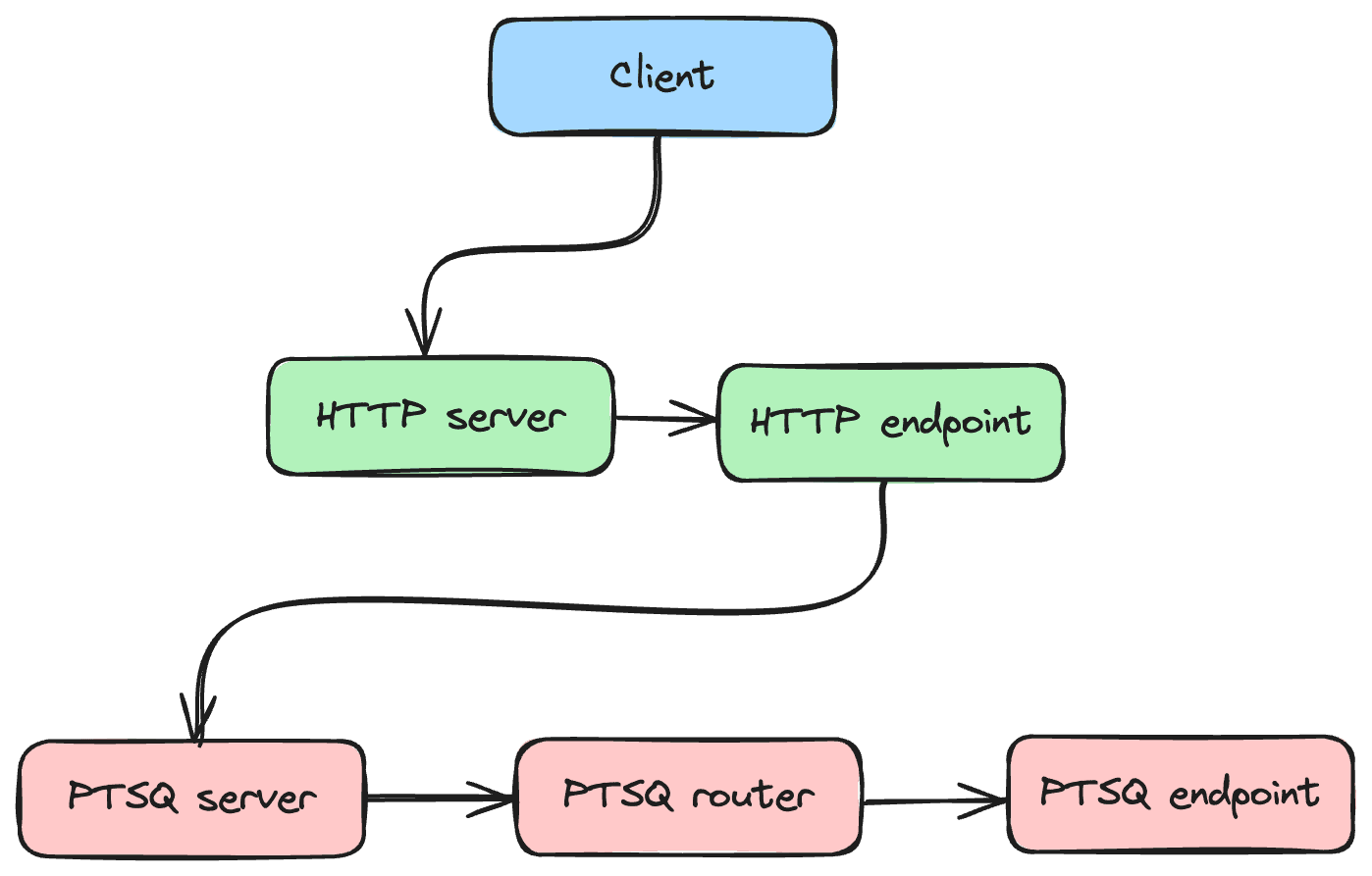
import { ptsq } from '@ptsq/server';
const { resolver, router serve, ptsqEndpoint } = ptsq().create();
You can directly export all created components for further use.
import { ptsq } from '@ptsq/server';
export const { resolver, router serve, ptsqEndpoint } = ptsq().create();
Server options
Context and CORS
Both context and CORS are described in other chapters.
Endpoint
The endpoint
setting specifies the path on which the PTSQ server will listen for requests.
The default is /ptsq
, which means that the server will listen on the HTTP endpoint /ptsq
, for example http://localhost:3000/ptsq
.
If the PTSQ server is deployed inside a REST API endpoint, for example /api
, the endpoint
setting must respect this and so its value must be set to /api/ptsq
.
This is especially necessary when using PTSQ with a full-stack framework such as Next.js or SvelteKit, where the application can be deployed in the file-system routers provided by these frameworks.
With this setting, trailing slashes are automatically removed, so it doesn't matter whether the value is set as /api/ptsq
or api/ptsq
.
ptsq({
//...,
endpoint: 'api/ptsq',
});
ptsq({
//...,
endpoint: '/api/ptsq',
});
fetchAPI
The fetchAPI option is for defining which API you will use for the request and response.
import type { Request, Response } from 'framework';
ptsq({
//...,
fetchAPI: {
Request: Request,
Response: Response,
},
});
It's very useful if you are using some integration that has its own request or response object.